ModelRight's Object Model: Background
Contents
Outline of sections below
- Background on ModelRight's Object Model
- A database model as captured in MRObjects
- Sample VBScript code to navigate the model
- Completing your understanding of the structure of ModelRight's data
Background on ModelRight's Object Model
Having covered some basic skills in operating ModelRight's programming environment, we move on to discussing what objects ModelRight exposes for your code to manipulate and how to access them.
In broad outline, ModelRight's Automation is much like Word and Excel: Your code starts with a reference to some top-level object, which provides a reference to collections of subsidiary objects, and each object has properties your code can read or write. You might therefore expect to proceed something like this:
'--- NOT QUITE how it works! ---
Set ATable = AModel.Tables("SomeTable")
Set AColumn = ATable.Columns("SpecialColumn")
AFieldLen = AColumn.Length
... and so on... |
Here,
- AModel.Tables and ATable.Columns would be a collection having children which are Table and Column objects respectively.
- AColumn.DataType would be a property of a Column object, setting or telling its data type.
ModelRight's data structure, while indeed capturing data of this type, is actually arranged more generally. Instead of specifically named collections, object types and properties, ModelRight's tree of model data is assembled mostly from objects of class MRObject each with a collection of children of class MRObject. To each MRObject is attached a collection of objects of class MRProperty which hold each specific property value. In aggregate, these hold data about models, tables, columns, views indexes and so on, as determined by the MRObject.Type field of each MRObject.
The rationale for the generic MRObjects stems from different vendor's databases having different features, requiring a modeling tool to maintain different collections and properties to capture the model's data for each database type. Rather than create a different internal data structure and automation model for each database vendor, ModelRight uses these generic MRObjects, and assembles the appropriate collections of children and properties as needed according to vendor-specific metamodel data, which we will meet below.
- The main features of MRObject are:
- A collection of child MRObjects
- A collection of MRProperties.
- An MRObject has a Type field, telling what role it plays in a ModelRight model, and a Name field. Example: Model info for a database table tblCustomers might be stored in an MRObject having MRObject.Name = "tblCustomers" and MRObject.Type = "Table".
- Methods to access children and properties conveniently, avoiding your code having to search through the Children and Properties collections explicitly.
- The main features of MRProperty (and helpers) are:
- A Name field that tells the name of the property
- Type field telling the data type of the property
- Value: the actual value
- Methods to set and retrieve the value from/to different data types
We will see how this plays out shortly, but the main impact on programming is that the above snippet of code looks more like the following:
'--- Using MRObjects ---
Set ATable = AModel.ChildByName("Table", "SomeTable")
Set AColumn = ATable.ChildByName("Column", "SpecialColumn")
AFieldLen = AColumn.Property("Datatype Length").AsInteger
... and so on... |
There are more variations for accessing children and properties, as can be seen in the ModelRight Object Reference. Also, writing to properties involves some additional steps, covered in a later section.
A database model as captured in MRObjects
Combining the above-described classes with a couple of additional classes gives us the following sketch of a typical database model, as contained in ModelRight objects:
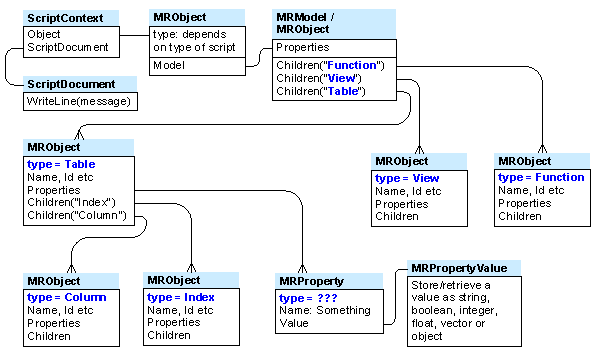
Things to note:
- Objects of the MRObject class appear in several roles. The MRObject.Type attribute tells whether it's describing a Table, View, Column, Index etc.
- MRObjects have a collection of children attached to them, which I've shown as a "Children" member, plus a crows-foot connector to imply multiple objects attached. This is shorthand for several functions to access children which you can see in the MRObject section of the ModelRight Objects Reference . Examples:
Set MyColumn = MyTable.AllChildren(1) |
Retrieves a single child MRObject (the first one, since the index is 1) which might contain information about a column, an index, or possibly something else. |
Set MyColumns = MyTable.Children("Column")
For each AColumn in MyColumns
' do something with AColumn
Next |
First retrieves a collection of child MRObjects of type "Column", then iterates through them. |
Set MyColumn =
MyTable.ChildByName("Column", "PersonId") |
Retrieves a single child MRObject whose Type = "Column" and having name "PersonId". |
Sample VBScript code to navigate the model
To consolidate this knowledge, here is a sample procedure which navigates a ModelRight model, picking out some basic info:
Sub Evaluate_OnLoad
Set Context = CreateObject("SCF.ScriptContext")
Set Document = Context.ScriptDocument
Set ThisScript = Context.Object
Set Model = ThisScript.Model
Document.WriteLine "Model: " & Model.Name
Set ModelMRObject = Model.AsObject
Document.WriteLine "ModelMRObject: " & ModelMRObject.Name
Set Tables = ModelMRObject.Children("Table")
Document.WriteLine "------ Tables and columns -------"
For Each Table In Tables
Set TableNameProp = Table.Property("Name")
TableName = TableNameProp.AsString
Document.WriteLine Table.TypeName & ": " & Table.Name
Set Columns = Table.Children("Column")
For Each Column in Columns
Document.WriteLine " " & TableName _
& "." & Column.Property("Name").AsString
_
& " : " & Column.Property("Datatype").AsString
Next
Next
end sub |
The statements involving Context and Model are often-used preliminary steps to gain initial access to the ModelRight programming interface. For more details on this see the ScriptContext and MRModel sections in the ModelRight Objects Reference.
The above script produces results like this (using the sakilla database model supplied with ModelRight MySQL):
Model: Model 1
ModelMRObject: Model 1
------ Tables and columns -------
Table: actor
actor.actor_id : SMALLINT
actor.first_name : VARCHAR(45)
actor.last_name : VARCHAR(45)
actor.last_update : TIMESTAMP
Table: address
address.address_id : SMALLINT
address.address : VARCHAR(50)
address.address2 : VARCHAR(50)
[...] |
Completing your understanding of the structure of ModelRight's data
Armed with the background just presented, let's summarize what areas of knowledge you will need in order to access ModelRight's data:
- The ModelRight COM object model: This is the subject we have just introduced, and it is further documented in the ModelRight Object Reference. Now you know that the classes MRObject and MRProperties are especially central, so examine these classes to closely.
- Initial reference: Understanding how your code should obtain an initial reference to a ModelRight object, through which to access the rest of the model. As in the example above, this entails objects ScriptFramework or ScriptContext, documented in the Object Reference.
- Understanding how database-model information, regarding tables, columns, views, indexes and so on, appear in the tree of MRObjects and MRProperties. The preceding example gives a taste, but to further develop this area we turn to ModelRight's browsing tools: Model Explorer, Model Browser, and Metamodel Browser. This is the subject of the next page.
Original article by Graham Wideman
|